Continuous Integration and Delivery for Monorepos
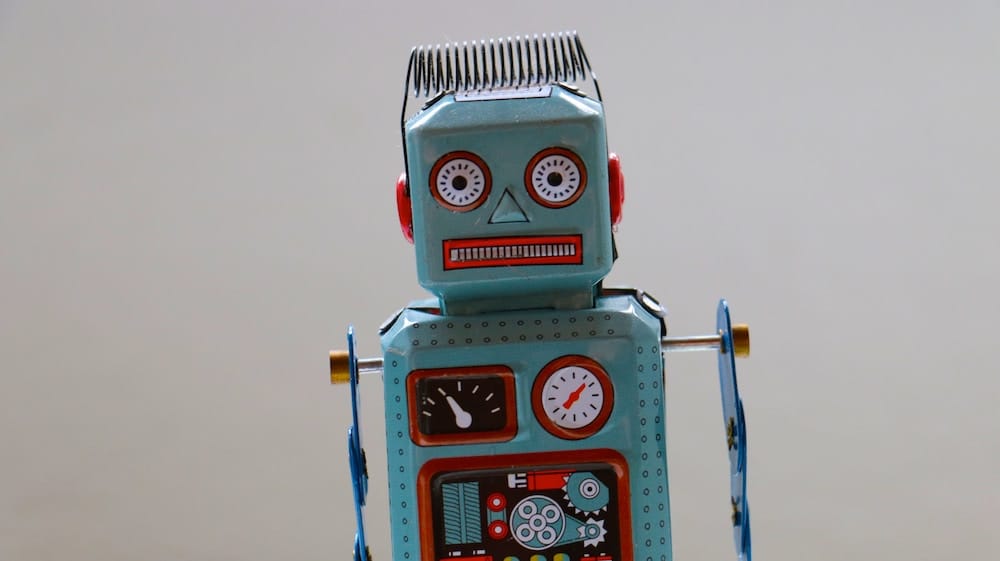
Recently I was working in a monorepo and wanted to set up continuous integration and devlivery for a bunch of microservices. I was surprised that I didn't find any popular library for this and a little excited about coding my own 🤓.
The basic idea is that if a change in the last git commit has happened in a service directory, we should trigger it's build.
Instead of running my build script directly I would let this bash script handle it (it can probably be improved in many ways 😅).
#!/bin/bash -e
build_changed() {
folders=$(git --no-pager diff --name-only HEAD~1 | sort -u | awk 'BEGIN {FS="/"} {print $1}' | uniq);
services="a_service another_service";
s=($(echo "$services" | tr ' ' '\n'));
f=($(echo "$folders" | tr ' ' '\n'));
for i in "${s[@]}"; do
for j in "${f[@]}"; do
if [[ $i = $j ]]; then
cd $i && ./bin/build.sh
cd ..
fi
done
done
}
build_changed
First we get the folders that are changed by the last commit. Then we intersect it with folders that contains services that are supposed to be built when changed, and run their respective build script 🎉.